Complex Numbers
Contents
Complex Numbers#
Many high school programs omit complex numbers, and some treatments even in university are bad (bad pedagogically and sometimes even bad logically). So it’s quite possible that a student in this course or a reader of this book will need an upgrade of their skills and understanding. Luckily, looked at the right way, the basics are very simple, and there is some amazing new pedagogy made possible by use of computer graphics.
We should point out that most of the hate directed at complex numbers is because arithmetic by hand is a bit more involved than that for real arithmetic, especially division. But, really, nobody has to do complex arithmetic by hand more than a few times to get the hang of it, anymore. The drudgery is quite usefully relegated to a computer.
Let’s begin by clearing out some logical `underbrush’ in order to clarify the issues. Plot
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(-2, 2, 50)
plt.grid()
plt.axhline(y=0, color='k')
plt.axvline(x=0, color='k')
plt.plot(x, x*x + 1)
plt.plot(x, x*0)
plt.xlim(-1.5,1.5)
plt.ylim(-1,4)
plt.show()
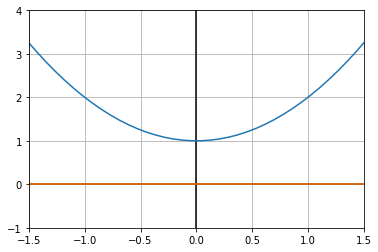
Very clearly there is no real
Because mathematicians use the words “real number” to mean numbers that are the limits of converging sequences of rational numbers (which gives a solid definition to them), and in the meantime the word “real” has an entirely different meaning in the “real world”, we have an unfortunate opportunity for miscommunication. The phrase “there is no real number which when squared gives
Even the word “number” has different meanings in math than in real life. If your partner says “I have a number of things to talk to you about” it certainly doesn’t mean they have nothing to say! Whereas to a mathematician, zero is a perfectly good number. The collision between math language and ordinary language really does cause problems sometimes.
This is quite unfortunate, because as we will now show concretely,
What is it? The complex number
The rules for adding and subtracting complex numbers (pairs of real numbers) are easy:
(just add or subtract component-wise, like vectors). Multiplication has a funny rule:
We will see shortly that this rule makes more sense in polar coordinates. For now, note that
At this point most people’s brains go, Click! “Why didn’t teachers just say this?” Well, two reasons. First, most people will just want to “get on with it” and go, and it all works, so why fuss? Second, it actually took a long time for this simple idea to be thought of. People were just saying things like “Well, let
Polar Coordinates for Complex Numbers#
When the famous physicist Richard Feynman talked about multiplication of complex numbers, he didn’t use the Cartesian rule
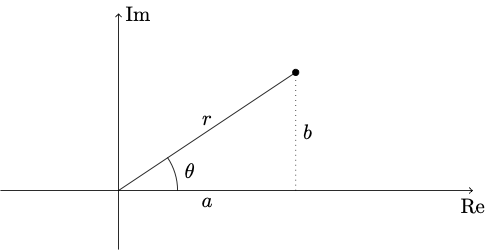
To get to the point
and by Pythagoras’ Theorem
Computing
and
Taking the ratio loses one important bit of information, namely the quadrant:
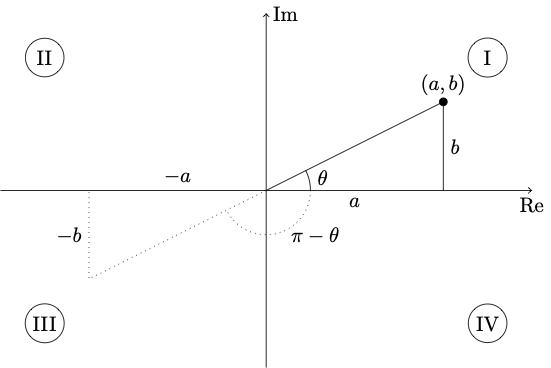
Since
Moreover, we take conventionally
Theorem 2 (The multiplication rule in polar coordinates)
If
That is,
and
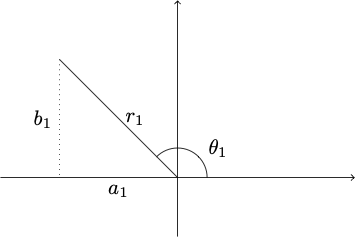
If
Proof. Use
and prove it yourself.
Example 1
Multiply
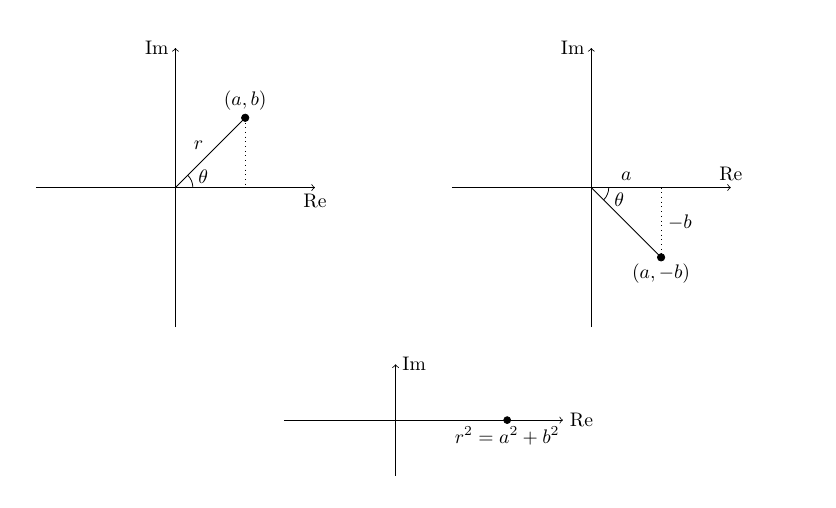
Pretty clearly the angle for
Therefore
which is a real nonnegative number!
Check in Cartesian:
just the same. The number
Note
If
Theorem 3 (Euler’s Theorem:
The notation
Then the multiplication rule fits the addition law of multiplying exponentials:
Why is
Another way is by differential equation:
and so one expects
so
which satisfies the same functional equation as
One can run this backwards, like so
because cosine is even and sine is odd.
so
These lovely formulae make trig identities simpler, like so:
Complex Weirdness#
Now that we have
So what?
…so for instance defining
Say what?
Well, if
and
if
… If you say so
But there’s more to this
for any integer
So, actually,
for any integer
because
Let’s use David Jeffrey’s notation and say
Therefore, we could have (but didn’t) define
an infinite number of possible real values. This isn’t the weirdest thing about complex numbers; but following the rules, it will all make sense, and some of it will be very useful indeed.
We just have to share an interesting new fact from Twitter. Christopher D. Long (@octonion) asked about the infinite tower of powers
and was surprised to learn that this thing has a known value (well, for a certain value of “known”). Actually, we were surprised, too, at how simple it turned out to be. The answer involves the Lambert W function: see The Lambert W Function Poster for a picture. The answer is that
To prove that, we have to carefully define that tower. We do so this way: put
from scipy import special as spc
import numpy as np
import math
c = 2.0j*spc.lambertw(math.pi/(2.0j))/math.pi
print(c)
N = 1000
xi = np.zeros(N,dtype=complex)
xi[0] = 0.0 # we already knew this
for k in range(N):
xi[k] = 1.0j**xi[k-1]
print(xi[-1])
print(abs(c - xi[-1])/abs(c))
(0.4382829367270321+0.3605924718713855j)
(0.4382829367270322+0.3605924718713855j)
1.9561499857674642e-16